Understanding GraphQL in 2025: A Comprehensive Guide
GraphQL fundamentals, best practices, and real-world implementation examples.
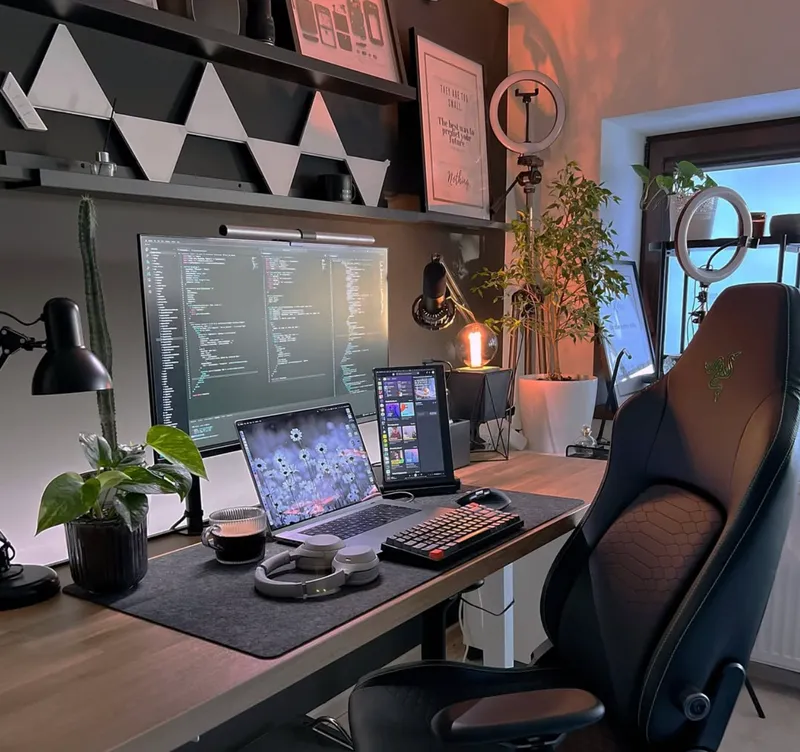
GraphQL has revolutionized how we think about API development and data fetching. Unlike traditional REST APIs, GraphQL puts the power in the hands of the client, allowing for precise data requests and efficient responses. This comprehensive guide will take you from the basics to advanced implementation patterns.
What Makes GraphQL Different? #
Think of GraphQL as a smart waiter at a restaurant. Instead of the kitchen (server) deciding what goes on your plate (like in REST APIs), you tell the waiter (GraphQL) exactly what you want. This means:
• No over-fetching: You get only what you ask for
• No under-fetching: You can get multiple resources in a single request
• Type safety: The schema defines exactly what's possible
• Efficient updates: The API can evolve without versioning
Core Concepts #
1. Schema DefinitionThe schema is your API's contract with clients. It defines what queries and mutations are possible:
scalar DateTime type User { id: ID! fullName: String! email: String! posts: [Post!]! createdAt: DateTime! } type Post { id: ID! title: String! content: String! author: User! tags: [String!] publishedAt: DateTime } type Query { users: [User!]! user(id: ID!): User posts(limit: Int = 10, offset: Int = 0): [Post!]! } type Mutation { createUser(input: CreateUserInput!): User! updateUser(id: ID!, input: UpdateUserInput!): User! deleteUser(id: ID!): Boolean! } input CreateUserInput { fullName: String! email: String! } input UpdateUserInput { fullName: String email: String
}
One of GraphQL's key strengths is its ability to evolve without versioning. Here's how you can safely evolve your schema:
# Get user details with their posts query GetUserWithPosts($userId: ID!) { user(id: $userId) { id fullName email posts { id title content publishedAt } }
}
This approach allows you to:
• Add new fields without breaking existing queries
• Deprecate old fields gracefully
• Provide clear migration paths for clients
• Maintain backward compatibility
3. Queries: Reading DataQueries in GraphQL are declarative and hierarchical:
# Get user details with their posts query GetUserWithPosts($userId: ID!) { user(id: $userId) { id fullName email posts { id title content publishedAt } }
}
Mutations follow similar patterns to queries but are used for creating, updating, or deleting data:
# Create a new user mutation CreateNewUser($input: CreateUserInput!) { createUser(input: $input) { id fullName email createdAt } } # Variables { "input": { "fullName": "John Doe", "email": "john@example.com" }
}
Real-World Implementation #
Setting Up a GraphQL ServerHere's a production-ready example using Apollo Server and TypeScript:
import { ApolloServer } from '@apollo/server'; import { startStandaloneServer } from '@apollo/server/standalone'; import { GraphQLError } from 'graphql'; import { PrismaClient } from '@prisma/client'; import DataLoader from 'dataloader'; // Initialize Prisma Client with logging in development const prisma = new PrismaClient({ log: process.env.NODE_ENV === 'development' ? ['query', 'error'] : ['error'], }); // Define the schema const typeDefs = #graphql scalar DateTime type User { id: ID! fullName: String! email: String! posts: [Post!]! createdAt: DateTime! } type Post { id: ID! title: String! content: String! author: User! publishedAt: DateTime } type Query { users: [User!]! user(id: ID!): User posts(limit: Int = 10, offset: Int = 0): [Post!]! } type Mutation { createUser(input: CreateUserInput!): User! updateUser(id: ID!, input: UpdateUserInput!): User! deleteUser(id: ID!): Boolean! } input CreateUserInput { fullName: String! email: String! } input UpdateUserInput { fullName: String email: String } ; // Create DataLoader for efficient data fetching const createLoaders = () => ({ userPosts: new DataLoader(async (userIds: readonly string[]) => { const posts = await prisma.post.findMany({ where: { authorId: { in: userIds as string[] } }, }); return userIds.map(id => posts.filter(post => post.authorId === id)); }), }); // Implement resolvers const resolvers = { Query: { users: async () => { try { return await prisma.user.findMany(); } catch (error) { throw new GraphQLError('Failed to fetch users', { extensions: { code: 'DATABASE_ERROR', error }, }); } }, user: async (_, { id }) => { try { const user = await prisma.user.findUnique({ where: { id }, }); if (!user) { throw new GraphQLError('User not found', { extensions: { code: 'NOT_FOUND' }, }); } return user; } catch (error) { throw new GraphQLError('Failed to fetch user', { extensions: { code: 'DATABASE_ERROR', error }, }); } }, posts: async (_, { limit = 10, offset = 0 }) => { try { return await prisma.post.findMany({ take: limit, skip: offset, orderBy: { publishedAt: 'desc' }, }); } catch (error) { throw new GraphQLError('Failed to fetch posts', { extensions: { code: 'DATABASE_ERROR', error }, }); } }, }, User: { posts: async (parent, _, { loaders }) => { try { return await loaders.userPosts.load(parent.id); } catch (error) { throw new GraphQLError('Failed to fetch user posts', { extensions: { code: 'DATABASE_ERROR', error }, }); } }, }, }; // Create and start the server const server = new ApolloServer({ typeDefs, resolvers, }); const { url } = await startStandaloneServer(server, { context: async () => ({ loaders: createLoaders(), }), listen: { port: 4000 }, });
console.log(🚀 Server ready at: ${url});
Here's how to consume the GraphQL API using Apollo Client in a React application:
import { ApolloClient, InMemoryCache, ApolloProvider, gql, useQuery } from '@apollo/client'; // Initialize Apollo Client const client = new ApolloClient({ uri: 'http://localhost:4000/graphql', cache: new InMemoryCache(), }); // Define your queries const GET_USERS = gql query GetUsers { users { id fullName email posts { id title } } } ; // Create a component function UserList() { const { loading, error, data } = useQuery(GET_USERS); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <div> <h2>Users</h2> <ul> {data.users.map(user => ( <li key={user.id}> <h3>{user.fullName}</h3> <p>{user.email}</p> <h4>Posts:</h4> <ul> {user.posts.map(post => ( <li key={post.id}>{post.title}</li> ))} </ul> </li> ))} </ul> </div> );
}
Subscriptions allow real-time data updates over WebSocket connections:
type Subscription { userStatusChanged(userId: ID!): UserStatus! newPost: Post! } type UserStatus { userId: ID! isOnline: Boolean! lastSeen: DateTime!
}
Implementation with Apollo Server:
import { WebSocketServer } from 'ws'; import { useServer } from 'graphql-ws/lib/use/ws'; import { makeExecutableSchema } from '@graphql-tools/schema'; const schema = makeExecutableSchema({ typeDefs, resolvers }); // Set up WebSocket server const wsServer = new WebSocketServer({ server: httpServer, path: '/graphql', }); // Configure WebSocket server const serverCleanup = useServer( { schema, context: async ctx => { // Add authentication here return { prisma, loaders: createLoaders() }; }, onConnect: async ctx => { // Handle connection console.log('Client connected'); }, }, wsServer ); // Add subscription resolvers const resolvers = { Subscription: { userStatusChanged: { subscribe: async function<em> (_, { userId }) { // Implementation of status monitoring while (true) { const status = await checkUserStatus(userId); yield { userStatusChanged: status }; await new Promise(resolve => setTimeout(resolve, 1000)); } }, }, newPost: { subscribe: (_, __, { pubsub }) => pubsub.asyncIterator(['POST_CREATED']), }, },
};
Handle file uploads using the graphql-upload
package:
import { GraphQLUpload } from 'graphql-upload'; const typeDefs = #graphql scalar Upload type File { filename: String! mimetype: String! encoding: String! url: String! } type Mutation { uploadFile(file: Upload!): File! updateUserAvatar(userId: ID!, avatar: Upload!): User! } ; const resolvers = { Upload: GraphQLUpload, Mutation: { uploadFile: async (_, { file }) => { const { createReadStream, filename, mimetype, encoding } = await file; // Process file upload const stream = createReadStream(); const path = /uploads/${Date.now()}_${filename}; await new Promise((resolve, reject) => { stream .pipe(createWriteStream(path)) .on('finish', resolve) .on('error', reject); }); return { filename, mimetype, encoding, url: https://your-domain.com${path}, }; }, },
};
Use schema directives to add rich documentation:
directive @deprecated( reason: String = "No longer supported" ) on FIELD_DEFINITION | ENUM_VALUE directive @auth(requires: Role = USER) on OBJECT | FIELD_DEFINITION directive @rateLimit(max: Int = 1000, window: String = "1m") on FIELD_DEFINITION enum Role { USER ADMIN } type User { id: ID! fullName: String! email: String! @auth(requires: ADMIN) posts: [Post!]! @rateLimit(max: 100, window: "1m")
}
Implement sophisticated error handling using union types:
union UserResult = User | UserNotFoundError | ValidationError type Query { user(id: ID!): UserResult! } type ValidationError { message: String! field: String } type UserNotFoundError { message: String! userId: ID! } # Resolver implementation const resolvers = { UserResult: { __resolveType(obj) { if (obj.email) return 'User'; if (obj.userId) return 'UserNotFoundError'; if (obj.field) return 'ValidationError'; return null; }, }, Query: { user: async (_, { id }) => { try { const user = await prisma.user.findUnique({ where: { id } }); if (!user) { return { __typename: 'UserNotFoundError', message: User with ID ${id} not found, userId: id, }; } return user; } catch (error) { return { __typename: 'ValidationError', message: error.message, field: error.field, }; } }, },
};
Implement comprehensive monitoring:
import { ApolloServer } from '@apollo/server'; import { ApolloServerPluginUsageReporting } from '@apollo/server/plugin/usageReporting'; import { ApolloServerPluginLandingPageLocalDefault } from '@apollo/server/plugin/landingPage/default'; import { PrometheusExporter } from '@opentelemetry/exporter-prometheus'; import { metrics } from '@opentelemetry/api-metrics'; // Set up monitoring plugins const server = new ApolloServer({ typeDefs, resolvers, plugins: [ // Usage reporting ApolloServerPluginUsageReporting({ sendTraces: true, sendVariableValues: { all: true }, }), // Metrics exporter { async requestDidStart() { const start = Date.now(); return { async willSendResponse(requestContext) { const duration = Date.now() - start; metrics.getMetric('graphql_operation_duration').record(duration, { operation: requestContext.operation?.operation || 'unknown', status: requestContext.errors?.length ? 'error' : 'success', }); }, }; }, }, // Development tools ApolloServerPluginLandingPageLocalDefault({ embed: true }), ], }); // Set up Prometheus metrics const prometheusExporter = new PrometheusExporter({ port: 9464, startServer: true, }); // Define custom metrics const operationDuration = metrics.createHistogram( 'graphql_operation_duration', { description: 'Duration of GraphQL operations', unit: 'ms', boundaries: [10, 50, 100, 200, 500, 1000], } ); // Example dashboard query (Grafana) const dashboardQuery = # Query operation durations rate(graphql_operation_duration_sum[5m]) / rate(graphql_operation_duration_count[5m])
;
Best Practices for 2025 #
- Use TypeScript
• Provides better type safety and developer experience
• Enables better IDE support and autocompletion
- Implement DataLoader
• Prevents N+1 query problems
• Batches and caches database queries efficiently
- Error Handling
• Use specific error codes and messages
• Include helpful error details in extensions
• Handle both expected and unexpected errors
- Schema Design
• Define custom scalars for specific types (e.g., DateTime)
• Use input types for mutations
• Implement pagination with sensible defaults
• Document fields with descriptions
- Security
• Implement proper authentication and authorization
• Validate input data
• Set appropriate rate limits
• Use HTTPS in production
- Performance
• Enable field-level caching where appropriate
• Implement query complexity analysis
• Monitor query performance
• Use persistent queries in production
- Testing
• Write schema tests
• Test resolvers independently
• Implement integration tests
• Use mocking for external dependencies
Resources and Tools #
Official Documentation Development Tools Libraries Production Deployment 1. Docker Configuration
FROM node:20-alpine WORKDIR /app COPY package</em>.json ./ RUN npm install COPY . . RUN npm run build EXPOSE 4000
CMD ["npm", "start"]
// config.ts import { config } from 'dotenv'; import { z } from 'zod'; config(); const envSchema = z.object({ NODE_ENV: z.enum(['development', 'production', 'test']), PORT: z.string().transform(Number), DATABASE_URL: z.string().url(), REDIS_URL: z.string().url(), JWT_SECRET: z.string().min(32), });
export const env = envSchema.parse(process.env);
const healthCheck = async () => { try { // Check database connection await prisma.$queryRawSELECT 1; // Check Redis connection await redis.ping(); return true; } catch (error) { console.error('Health check failed:', error); return false; } }; app.get('/health', async (req, res) => { const isHealthy = await healthCheck(); res.status(isHealthy ? 200 : 503).json({ status: isHealthy ? 'healthy' : 'unhealthy', timestamp: new Date().toISOString(), });
});
Conclusion #
GraphQL is a powerful tool that gives frontend developers more control over data fetching while making APIs more efficient and flexible. Start small, focus on the basics, and gradually incorporate more advanced features as you become comfortable with the fundamentals.
The key to successful GraphQL implementation is understanding your data requirements and designing a schema that reflects your domain model while being flexible enough to evolve with your application's needs.